/* Name: Program to convert Celsius to Farenheit
Author: Parveen Malik
Date: 06/01/11 15:36*/
#include<stdio.h>
#include<conio.h>
void main()
{
int lower,upper,steps;
int celsius;
float farn;
lower=0;
upper=100; //you can chanage these parameters like lower,upper and steps
steps=5;
celsius=lower;
printf("Celsius\t\tFarenheit\n\n"); // /t tab
while(celsius<=upper)
{
farn=((9.0/5.0)*celsius)+32.0; // formula to Celsius to Farenheit
printf("%d\t==>\t%.2f\n",celsius,farn);
celsius+=steps;
}
getch();
}
[caption id="attachment_156" align="aligncenter" width="655" caption="OUTPUT"]
[/caption]
Author: Parveen Malik
Date: 06/01/11 15:36*/
#include<stdio.h>
#include<conio.h>
void main()
{
int lower,upper,steps;
int celsius;
float farn;
lower=0;
upper=100; //you can chanage these parameters like lower,upper and steps
steps=5;
celsius=lower;
printf("Celsius\t\tFarenheit\n\n"); // /t tab
while(celsius<=upper)
{
farn=((9.0/5.0)*celsius)+32.0; // formula to Celsius to Farenheit
printf("%d\t==>\t%.2f\n",celsius,farn);
celsius+=steps;
}
getch();
}
[caption id="attachment_156" align="aligncenter" width="655" caption="OUTPUT"]
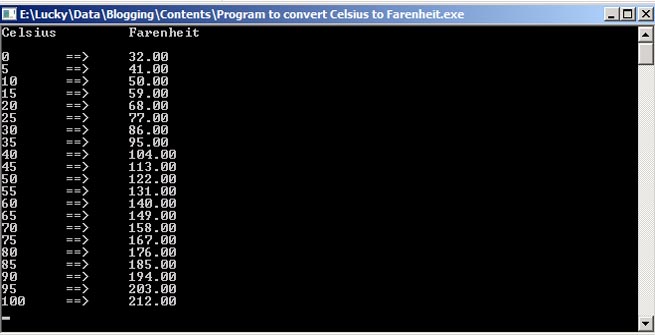